Spring Security – UserDetailsService and UserDetails with Example
Spring Security is a framework that allows a programmer to use JEE components to set security limitations on Spring-framework-based Web applications. In a nutshell, it’s a library that can be utilized and customized to suit the demands of the programmer. Read more on Spring Security and its Features in this article Introduction to Spring Security and its Features . UserDetails and UserDetailsService are two major concepts to learn in Spring Security. So we will learn these two concepts with proper examples.
UserDetailsService and UserDetails
In Spring Security, the UserDetailsService interface is a core component used for loading user-specific data. It is responsible for retrieving user information from a backend data source, such as a database or an external service, and returning an instance of the UserDetails interface.
The UserDetailsService interface has a single method called loadUserByUsername() , which takes a username as a parameter and returns a fully populated UserDetails object. The UserDetails object represents the authenticated user in the Spring Security framework and contains details such as the user’s username, password, authorities (roles), and additional attributes.
In this article, we are going to use
- InMemoryUserDetailsManager class , which is an implementation of the UserDetailsService interface that stores user details in memory. It is commonly used for testing and development purposes when you want to define user accounts directly in your code without the need for a persistent data store, and
- User class , which is a convenient builder class provided by the framework for creating instances of UserDetails. It is used to represent user account details, such as the username, password, and authorities (roles) associated with a user. The User class provides a fluent API for constructing UserDetails objects with various attributes. It offers methods to set the username, password, and roles for a user, among other properties.
So we are going to create the user with the help of both InMemoryUserDetailsManager and User class. A sample code is given below
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception
InMemoryUserDetailsManager userDetailsManager = new InMemoryUserDetailsManager();
// Method 1 - Creating username, password, and role
ArrayList roles = new ArrayList();
SimpleGrantedAuthority role1 = new SimpleGrantedAuthority("USER");
SimpleGrantedAuthority role2 = new SimpleGrantedAuthority("ADMIN");
roles.add(role1);
roles.add(role2);
User anshulUser = new User("anshul", "123", roles);
// Method 2 - Creating username, password, and role
// UserDetails anshulUser = User.withUsername("Anshul").password("123").roles("USER", "ADMIN").build();
userDetailsManager.createUser(anshulUser);
auth.userDetailsService(userDetailsManager);
>
Example Project
We’re going to build on top of the simple Spring MVC example.
Step 1: Create Your Project and Configure Apache Tomcat Server
Note : We are going to use Spring Tool Suite 4 IDE for this project. Please refer to this article to install STS in your local machine How to Download and Install Spring Tool Suite (Spring Tools 4 for Eclipse) IDE .
- Create a Dynamic Web Project in your STS IDE. You may refer to this article to create a Dynamic Web Project in STS: How to Create a Dynamic Web Project in Spring Tool Suite?
- Configure Apache Tomcat Server and configure the Tomcat Server with the application. Now we are ready to go.
Step 2: Folder Structure
Before moving to the project let’s have a look at the complete project structure for our Spring MVC application.
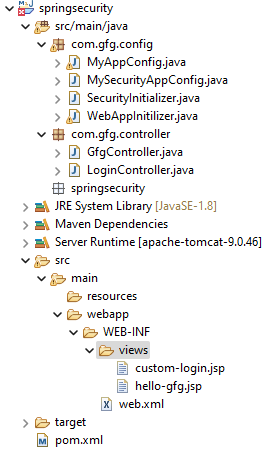
Step 3: Add Dependencies to pom.xml File
Add the following dependencies to your pom.xml file
- Spring Web MVC
- Java Servlet API
- Spring Security Config
- Spring Security Web